The Development of Moonsters
With Moonsters we wanted to create a game that is easy accessible and enjoyable to many people. The popular 'match 3' genre was a good choice to reach a large audience. This genre also limits the resources needed for development which is important for a studio of two persons.
We decided to monetize our game using 'in app purchase' even if this is not a popular model to everybody out there. A big plus for a 'free to play' app is that you can access it without a payment barrier. Even if the game would not be a financial success, we would be able to show it everybody.
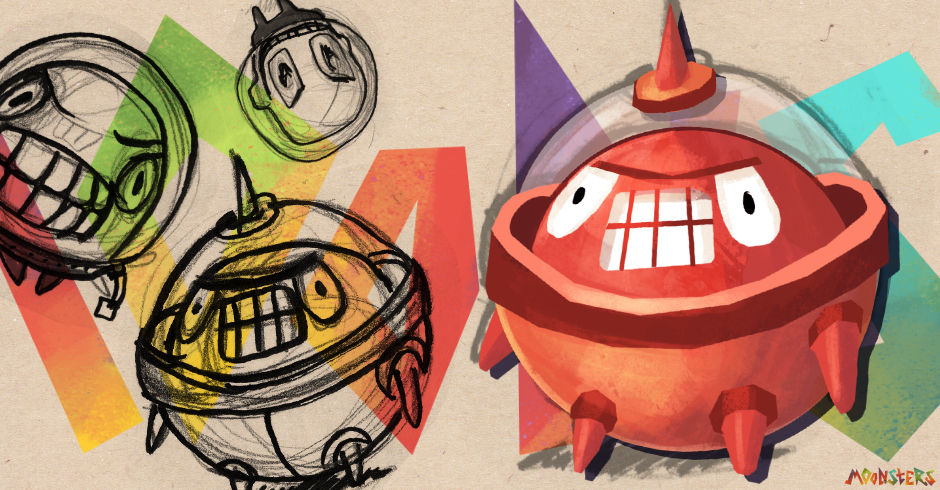
One challenge of the 'free to play' concept is the balancing of the game. We wanted to provide enough gameplay for people that are not willed to pay anything. There should also be a chance to play the whole game without a single purchase. At the same time there should be the option to buy helpful features to have a faster progress. The player can unlock the games features 'bomb', 'joker' and 'slingshot' by playing or by purchase. We think this is a fair approach.
In Moonsters you can improve your results if you play the same planet again. The best result will be saved for continuation. A good strategy is to replay earlier planets after you unlocked a feature.
Creative Stuff
For a 'match 3' you want to easily distinguish between the entities. That's why we designed every Moonster in a different shape. It took us some time to find the best look and tint for the Moonsters and their galaxy. Finally we came up with a style that is cute for children and droll for older players.
Another challenging part was the creation of the walls. We wanted them to have different damage models to give the player a good feedback of what he is doing. The wall should be constructed out of several parts to create different combinations.

The music was composed with the idea to create a comic-like sound for the game. It should enhance the satisfaction if you reach a goal in the game. Every feature did get its own sound to make it more unique. The music and sounds where created using Ableton Live and Reason.
The sound that you hear if you complete a planet.Technical Stuff
The game is written in Java using libGDX as the cross platform technology. libGDX uses OpenGL ES 2.0 to render the graphics and RoboVM to get Java run on iOS.
A great benefit of libGDX is that you can develop almost the whole game on your desktop machine. This saves the time for starting up an emulator or deploying to a real device. libGDX also gives you a good project structure with a core module for the game. The different platforms like android and iOS get their own modules. You can write the core game functionality in its module and let the platforms depend on it.
Platform specific code
When it comes to platform specific code it is a good idea to create interfaces for the needed functionality. In our case it was an interface for the payment, for social networks and for game services. If you designed your interfaces you can implement the logic for the platform in its own module.
For Moonsters we use Google Play Game Services to save the game progress on the cloud. This enables the player to share the progress between devices. At the end I do not think it was worth implementing it. It took longer than expected, annoys the player with a login and requires an internet connection.
Of cause we had to implement logic for the two payment APIs (Apple / Google). Fortunately the processes are not that different. Using the same identifiers for the purchase-entries on iTunes Connect and Google Developer Console was helpful. For iOS we used a so called RoboVM binding to get access to Apples purchase system. For Google we where able to use its standard APIs since they are using Java natively.
- Game Helper for the Google Game Services
- RoboVM binding for in-app-purchases (at least until iOS 9)
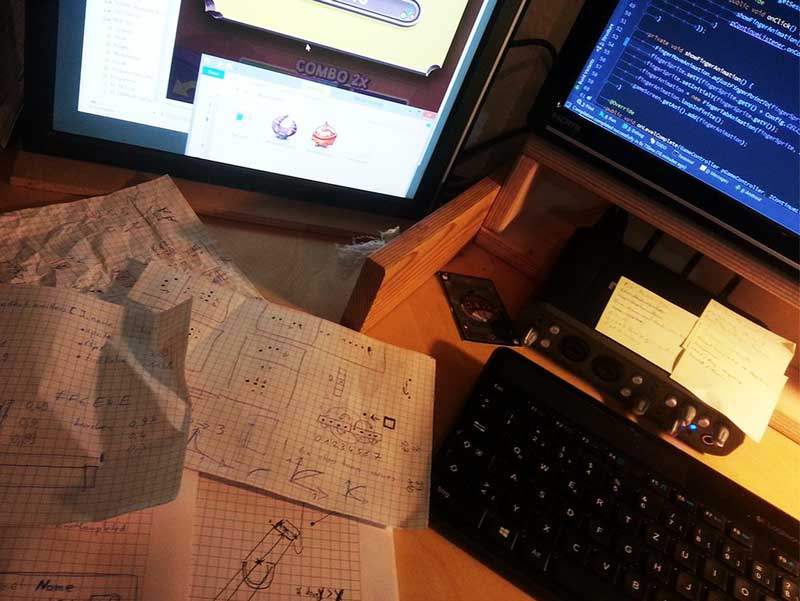
Animations
Moonsters is a game that has many animations combined with nice particle effects. We wrote a simple animation library to create animations exactly the way we wanted them. This library is called Ani and it is available on GitHub under a MIT License. It does not depend on other technologies and can be used for all Java applications. Only a few lines of code are needed to set it up, instantiate an animation and run it.
// Create an animation controller with an update interval of 30 milliseconds.
Ani ani = new Ani(30);
// Create an instance of our animation.
SimpleFadeAnimation myAnimation = new SimpleFadeAnimation(myGraphic);
// Add the animation.
ani.add(myAnimation);
To write a custom animation just extend the BaseAnimation class and implement your animation logic.
/**
* An animation to fade in a Graphic.
*/
public static class SimpleFadeAnimation extends BaseAnimation {
private Graphic graphic;
public SimpleFadeAnimation(Graphic pGrapic) {
super(5000); // sets the duration of 5 sec
graphic = pGraphic;
}
// called constantly while animating
@Override
public void onProgress(float progress) {
graphic.setAlpha(progress) // progress from 0.0 to 1.0
}
}
Beside Ani we used a bone animation editor called Spine. This was necessary to animate the walk, posing and surrender of the 'bad robot'. Spine is a handy tool that focuses on the stuff you really need. It exports animations for many available game frameworks like Unity, Cocos2d, XNA etc.
Used technologies
This is a list of technologies that we used for the development of Moonsters.
- libGDX game development framework
- RoboVM ahead-of-time Java compiler for iOS
- Ani Java animation library
- Spine Animation tool
- Gradle build automation
- Audacity audio recorder and editor
- IntelliJ Community Edition IDE
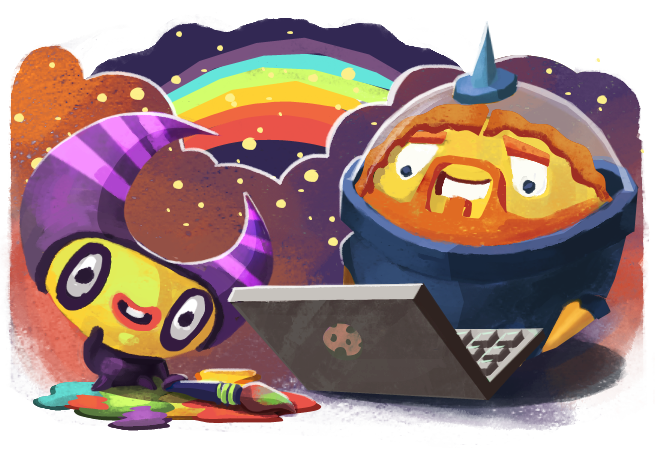